Managing Stored Procedures with Entity Framework Core Migrations in .NET 8
Author
Mohammad Ashiqul Islam
Date Published
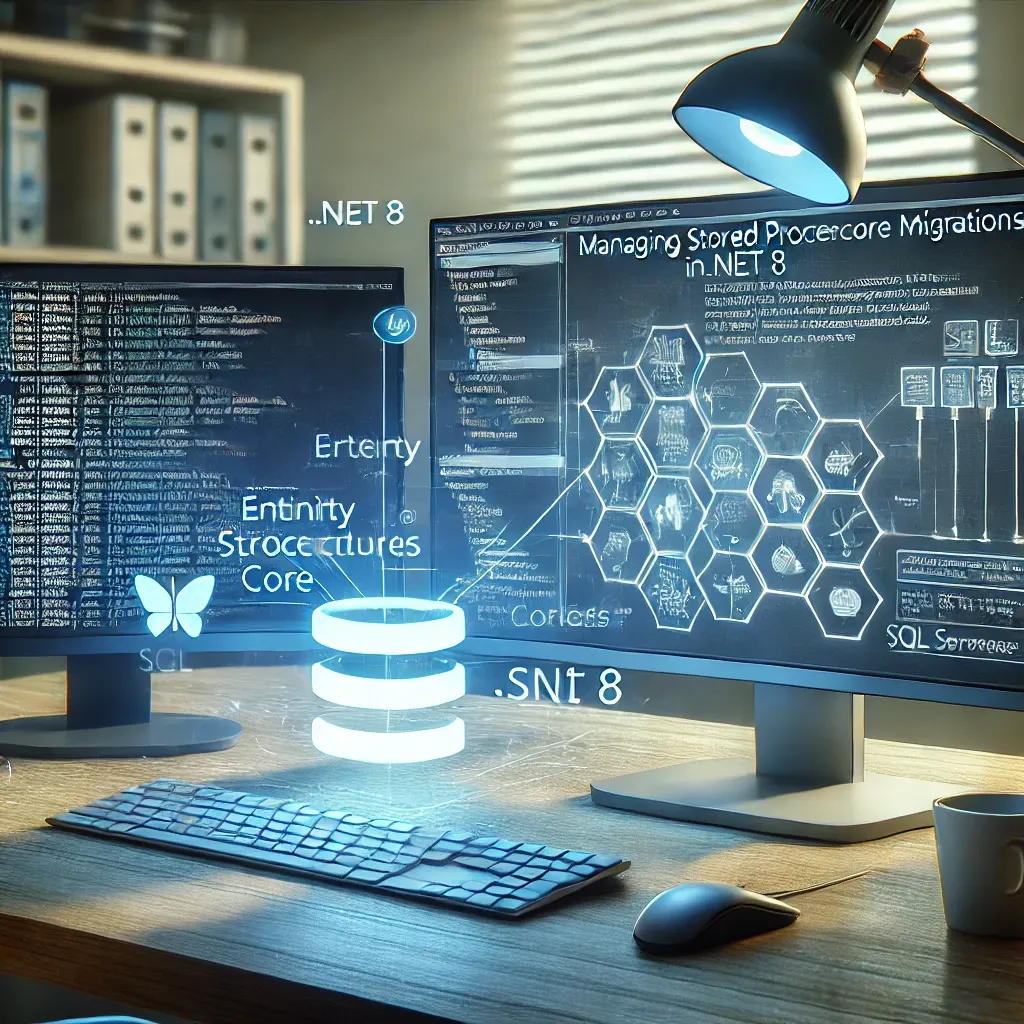
Entity Framework Core (EF Core) offers a robust way to manage your database schema using the Code First approach. With .NET 8, the process of handling stored procedures in EF Core migrations remains straightforward and effective. In this post, I’ll guide you through incorporating stored procedures as part of your migrations.
Why Use Stored Procedures?
Stored procedures can optimize complex database operations, encapsulate logic for reuse, and improve maintainability. With EF Core, while you can call stored procedures using methods like FromSqlInterpolated, there’s no built-in support to define and create them directly in code. However, we can use EF Core migrations to manage stored procedures effectively.
Step-by-Step Guide
1. Create an Empty Migration
To begin, generate an empty migration to house the stored procedure.
1dotnet ef migrations add GetOutboxMessages
This command generates a migration file with empty Up and Down methods.
2. Define the Stored Procedure in the Migration
Modify the Up and Down methods of the generated migration to create and drop the stored procedure, respectively.
1using Microsoft.EntityFrameworkCore.Migrations;23namespace YourNamespace.Migrations4{5 public partial class GetOutboxMessages : Migration6 {7 protected override void Up(MigrationBuilder migrationBuilder)8 {9 const string sp =10 """11 CREATE PROCEDURE [dbo].[GetOutboxMessages]12 @BatchSize INT13 AS14 SELECT TOP (@BatchSize) Id, Content15 FROM OutboxMessages WITH (UPDLOCK, READPAST)16 WHERE ProcessedOnUtc IS NULL17 ORDER BY OccurredOnUtc;18 """;1920 migrationBuilder.Sql(sp);21 }2223 protected override void Down(MigrationBuilder migrationBuilder)24 {25 migrationBuilder.Sql("DROP PROCEDURE [dbo].[GetOutboxMessages]");26 }27 }28}
Key Updates for .NET 8:
Improved SQL Syntax Support: Ensure your SQL scripts align with any database-specific features introduced in .NET 8 compatibility.
Enhanced Logging: Use EF Core’s enhanced logging features to debug stored procedure execution during migrations.
3. Apply the Migration
Run the following command to update the database schema and create the stored procedure.
1dotnet ef database update
This will execute the Up method and create the stored procedure in your database.
Conclusion
Using EF Core migrations to manage stored procedures provides a seamless way to version and deploy database logic. With .NET 8, the process remains powerful and integrates well with modern development practices. By including stored procedures in your migrations, you can ensure consistency across environments and streamline your deployment workflows.
Happy coding!